INTERFACE AND APPLICATION PROGRAMMING
GOALS FOR THE WEEK
- Compare as many tool options as possible.
- Write an application that interfaces a user with an input and/or output device that you made
GROUP
INDIVIDUAL
Link to the group assignment: TEST
CONCEPT
I wanted to create and interface for the a board I made during output week. It is a simple board with a servo attached to it.
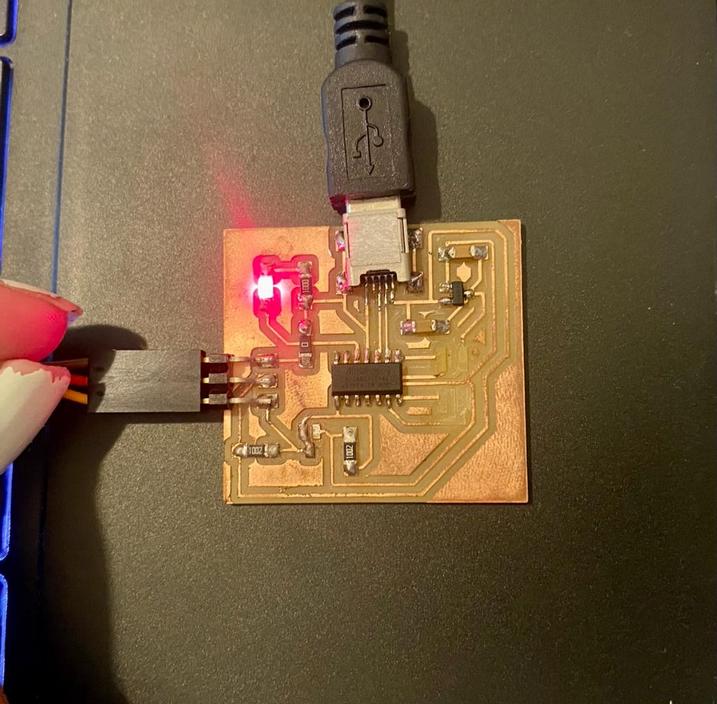
First I wrote a code in arduino that would let me control the movement of the servo using command interface. I also had to find the range of motion and values that work that servo and replicate the same range in processing.
The code in arduino
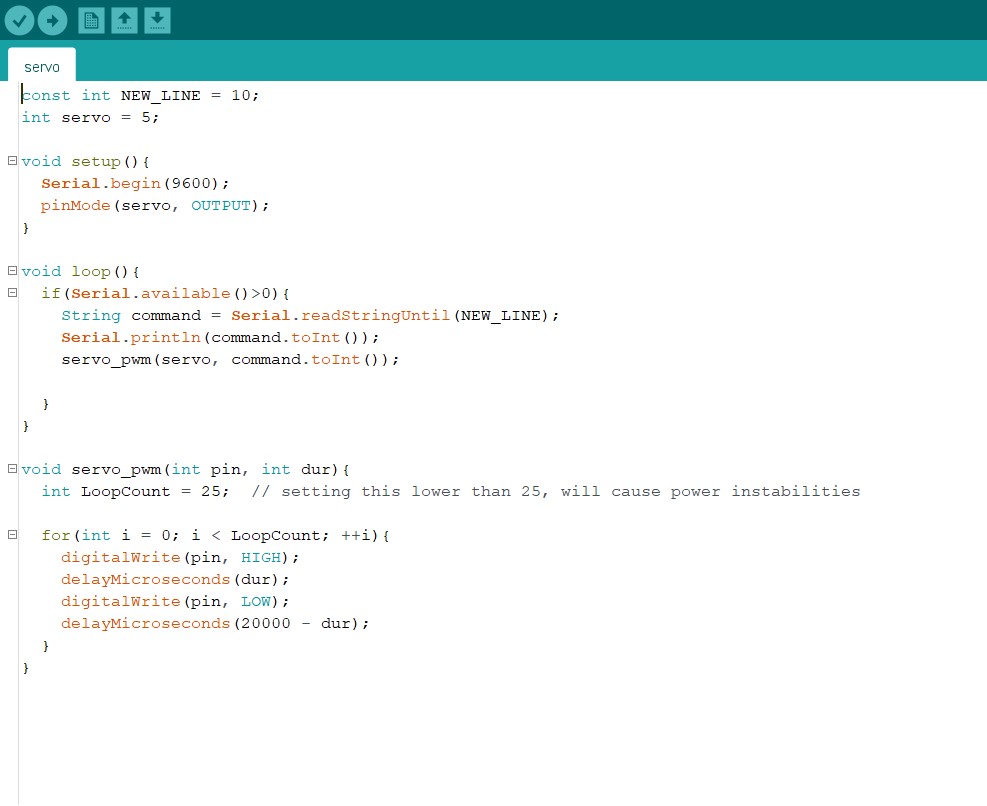
The goal this week in processing is to have a slider interface that can communicate angles to a servo.
PROCESSING
Processing is a flexible software sketchbook and a language for learning how to code within the context of the visual arts. Since 2001, Processing has promoted software literacy within the visual arts and visual literacy within technology. There are tens of thousands of students, artists, designers, researchers, and hobbyists who use Processing for learning and prototyping.
- It is an open source software and can be downloaded from processing.org.
- Processing is a great source for creating graphics. The Processing IDE works for a computer like the Arduino IDE works for a micro-controller. The Processing IDE is similar to Arduino in terms of structure.
- It has setup functions and draw functions like an Arduino has a setup and loop function.
- The Processing IDE can communicate with the Arduino IDE through serial communication. This way, we can send data from the Arduino to the Processing IDE and also from the Processing IDE to the Arduino.
BASIC PROCESSING COMMANDS
setup() : Like the setup() function in arduino, runs only once.
draw() : Updates the GUI, runs continuously like the kinda arduino loop() function.
keyPressed() : Event initiated when a keystroke is detected.
size(width,height) : Defines the dimension of the display window width and height in units of pixels.
background(rgb) : The background() function sets the color used for the background of the Processing window.
exit() : Quits/stops/exits the program. Programs without a draw() function stop automatically after the last line has run
Serial(parent, portName, baudRate) : Initiates a serial port for communication. The sketch will freeze if port is not found. It can be avoided by wrapping the entire section in a try block.
Serial.list(): Returns a string array of names of all the serial objects connected to the machine.
serialEvent(Serial obj) : This event is triggered everytime a serial event occurs. It may be when serial data is received.
serial.read() : Returns a number between 0 and 255 for the next byte that’s waiting in the buffer. Returns -1 if there is no byte, although this should be avoided by first cheacking available() to see if data is available.
serial.write() : Writes bytes, chars, ints, bytes[], Strings to the serial port
Code in processing
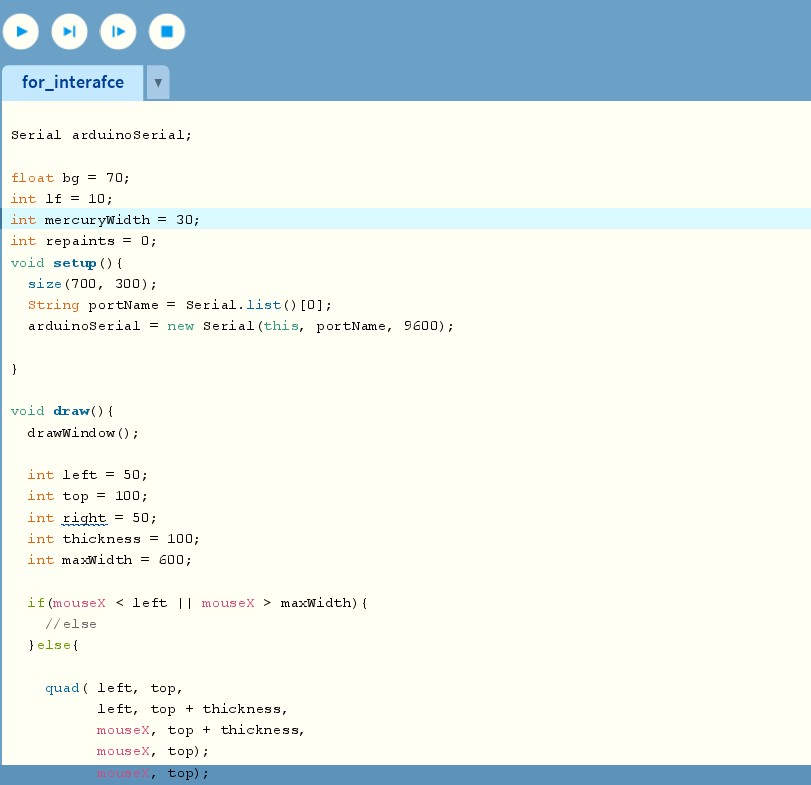
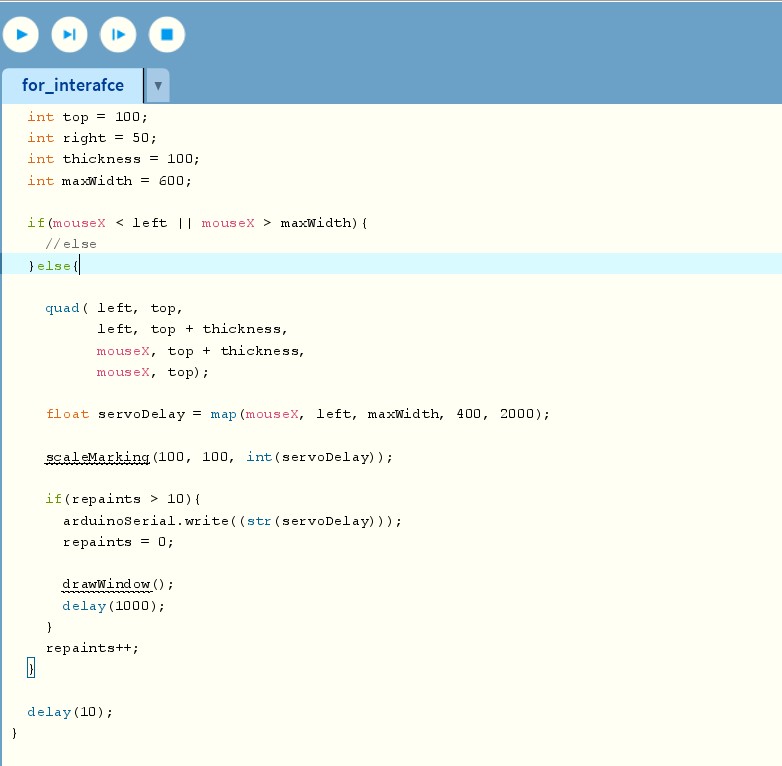
COMMUNICATION
- mousex: The rectangle is made by the movement of the mouse.
- map(): The range of the commands given to the servo in arduino is defined as the extent of the slider in processing(200-4000).
- maxwidth: There is nothing sent when the mouse in close to the borders.
- The command is sent only every 200 values.
Design files download
Arduino Code
Processing Code